Custom Cluster Metrics Autoscaling in Amazon EKS
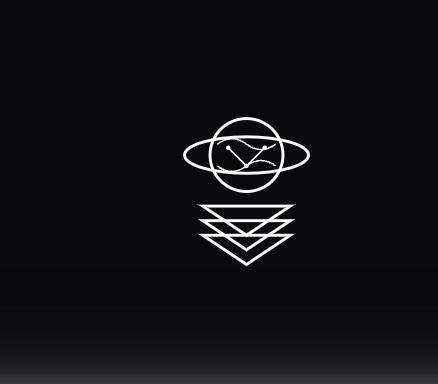
Mastering Custom Cluster Metrics Autoscaling in Amazon EKS: A Comprehensive Guide
In the ever-evolving landscape of cloud-native applications, the ability to efficiently manage resources is not just a luxury—it’s a necessity. As senior engineers, we’re often tasked with optimizing complex systems to ensure they’re both performant and cost-effective. Amazon Elastic Kubernetes Service (EKS) provides a robust platform for running containerized workloads, but its true power lies in how we leverage its features to create responsive, scalable systems. In this comprehensive guide, we’ll dive deep into implementing custom metrics autoscaling in Amazon EKS, equipping you with the knowledge to architect solutions that dynamically adapt to varying workloads.
Table of Contents
- Introduction to Kubernetes and EKS
- The Imperative of Autoscaling
- Kubernetes Autoscaling: A Deep Dive
- Custom Metrics Autoscaling: Beyond the Basics
- Implementing Custom Metrics Autoscaling in EKS: A Step-by-Step Guide
- Advanced Go Application Demo
- Comprehensive Load Testing with k6
- Best Practices and Architectural Considerations
- Troubleshooting and Performance Tuning
- Future-proofing Your Autoscaling Strategy
- Conclusion
Introduction to Kubernetes and EKS
Kubernetes has fundamentally transformed the container orchestration landscape, offering a declarative approach to application deployment and management. Its power lies in its ability to abstract away infrastructure complexities, allowing developers to focus on application logic rather than operational intricacies. Amazon EKS takes this a step further by providing a managed Kubernetes service, effectively eliminating the operational overhead of maintaining the Kubernetes control plane.
To truly appreciate the role of EKS in our autoscaling journey, let’s visualize its architecture:
+-----------------------------------------------------+
| AWS Cloud |
| +------------------------------------------------+ |
| | EKS Cluster | |
| | +--------------------+ +-------------------+ | |
| | | Control Plane | | Worker Nodes | | |
| | | +--------------+ | | +--------------+ | | |
| | | | API Server | | | | Kubelet | | | |
| | | +--------------+ | | +--------------+ | | |
| | | | etcd | | | | Container | | | |
| | | +--------------+ | | | Runtime | | | |
| | | | Scheduler | | | +--------------+ | | |
| | | +--------------+ | | | Pods | | | |
| | | | Controllers | | | +--------------+ | | |
| | | +--------------+ | | | Node Agents | | | |
| | | | | +--------------+ | | |
| | +--------------------+ +-------------------+ | |
| | ^ ^ | |
| | | | | |
| | v v | |
| +------------------------------------------------+ |
| ^ ^ |
| | | |
| v v |
| +------------------------------------------------+ |
| | AWS Services (ELB, EBS, VPC, IAM, etc.) | |
| +------------------------------------------------+ |
+-----------------------------------------------------+
This architecture illustrates the separation of concerns between the control plane (managed by AWS) and the worker nodes (where our applications run). This separation is crucial for understanding how autoscaling decisions are made and implemented.
The Imperative of Autoscaling
In the realm of cloud computing, the only constant is change. Traffic patterns fluctuate based on a myriad of factors: time of day, marketing campaigns, viral content, or even global events. This variability presents a fundamental challenge: how do we ensure our applications have sufficient resources to handle peak loads without over-provisioning during quieter periods?
This is where autoscaling becomes not just useful, but imperative. Let’s break down the multifaceted benefits of implementing a robust autoscaling strategy:
-
Cost Optimization: In a pay-per-use cloud model, running at peak capacity 24/7 is financially untenable. Autoscaling allows us to align our resource consumption with actual demand, potentially leading to significant cost savings.
-
Performance Improvement: By automatically scaling up during high-demand periods, we ensure that our application remains responsive, maintaining a consistent user experience even under increased load.
-
Resilience: Autoscaling contributes to system resilience by automatically replacing failed nodes or pods, effectively implementing a self-healing mechanism.
-
Efficient Resource Utilization: It helps in making optimal use of available resources, preventing both under-utilization (wasted resources) and over-utilization (performance bottlenecks).
-
Handling Unpredictable Loads: For applications with variable or unpredictable traffic patterns, autoscaling provides the flexibility to handle sudden spikes without manual intervention.
-
Environmental Benefits: By optimizing resource usage, autoscaling can contribute to reduced energy consumption in data centers, aligning with green computing initiatives.
To illustrate the impact of autoscaling, consider the following comparison:
Resource
Usage
^
| /\ /\
| / \ / \ /\
| / \ / \ / \
| / \/ \/ \
|/ \
+--------------------------> Time
Autoscaled Resources
Resource
Usage
^
|------------------------
| | Wasted Resources
| /\ /\ |
| / \ / \ /\ |
| / \ / \ / \ |
| / \/ \/ \|
|/ \
+--------------------------> Time
Fixed Resources
This visualization underscores the efficiency gains of autoscaling. The top graph shows how autoscaled resources closely follow actual resource usage, while the bottom graph illustrates how fixed resources often lead to either resource waste or capacity shortfalls.
Kubernetes Autoscaling: A Deep Dive
Kubernetes provides several built-in autoscaling mechanisms, each designed to address different scaling needs. Understanding these mechanisms is crucial for implementing an effective autoscaling strategy.
1. Horizontal Pod Autoscaler (HPA)
The HPA automatically scales the number of pods in a deployment, replication controller, or replica set based on observed CPU utilization or custom metrics.
How it works:
- The HPA controller periodically checks the metrics server for resource utilization.
- It calculates the desired number of replicas based on the current utilization and the target utilization.
- If the desired state differs from the current state, it updates the number of replicas.
When to use:
- For applications that can be scaled by adding more instances.
- When you want to scale based on CPU usage or custom metrics.
Example HPA configuration:
apiVersion: autoscaling/v2beta1
kind: HorizontalPodAutoscaler
metadata:
name: myapp-hpa
spec:
scaleTargetRef:
apiVersion: apps/v1
kind: Deployment
name: myapp
minReplicas: 2
maxReplicas: 10
metrics:
- type: Resource
resource:
name: cpu
targetAverageUtilization: 50
This configuration will maintain CPU utilization around 50% by scaling between 2 and 10 replicas.
2. Vertical Pod Autoscaler (VPA)
The VPA automatically adjusts the CPU and memory reservations for your pods to help “right size” your applications.
How it works:
- The VPA continuously monitors the resource usage of your pods.
- It recommends (or automatically applies) updated resource requests based on the observed usage.
- For pods that need to be updated, it can evict them so they’re rescheduled with the new resource requirements.
When to use:
- For applications that can’t be easily horizontally scaled.
- When you want to ensure pods have the right amount of resources without manual tuning.
Example VPA configuration:
apiVersion: autoscaling.k8s.io/v1
kind: VerticalPodAutoscaler
metadata:
name: myapp-vpa
spec:
targetRef:
apiVersion: "apps/v1"
kind: Deployment
name: myapp
updatePolicy:
updateMode: "Auto"
This configuration will automatically adjust the resource requests for the myapp
deployment based on observed usage.
3. Cluster Autoscaler
The Cluster Autoscaler automatically adjusts the number of nodes in your cluster when pods fail to schedule due to resource constraints.
How it works:
- It monitors for pods that can’t be scheduled due to insufficient cluster capacity.
- When it detects such pods, it increases the size of the node group.
- It also regularly checks for underutilized nodes and removes them if possible.
When to use:
- When you want to automatically adjust the size of your Kubernetes cluster based on workload.
- To ensure you have enough nodes to run all scheduled pods while minimizing costs.
Example Cluster Autoscaler configuration for AWS:
apiVersion: apps/v1
kind: Deployment
metadata:
name: cluster-autoscaler
namespace: kube-system
labels:
app: cluster-autoscaler
spec:
replicas: 1
selector:
matchLabels:
app: cluster-autoscaler
template:
metadata:
labels:
app: cluster-autoscaler
spec:
serviceAccountName: cluster-autoscaler
containers:
- image: k8s.gcr.io/cluster-autoscaler:v1.21.0
name: cluster-autoscaler
command:
- ./cluster-autoscaler
- --v=4
- --stderrthreshold=info
- --cloud-provider=aws
- --skip-nodes-with-local-storage=false
- --skip-nodes-with-system-pods=false
- --balance-similar-node-groups
- --expander=least-waste
- --node-group-auto-discovery=asg:tag=k8s.io/cluster-autoscaler/enabled,k8s.io/cluster-autoscaler/<YOUR_CLUSTER_NAME>
volumeMounts:
- name: ssl-certs
mountPath: /etc/ssl/certs/ca-certificates.crt
readOnly: true
volumes:
- name: ssl-certs
hostPath:
path: "/etc/ssl/certs/ca-bundle.crt"
This configuration sets up the Cluster Autoscaler to work with AWS Auto Scaling Groups (ASGs) tagged appropriately for your EKS cluster.
4 Custom Metrics Autoscaling: Beyond the Basics
While the built-in autoscalers are powerful, they may not always be sufficient for complex, real-world scenarios. This is where custom metrics autoscaling comes into play, allowing you to scale based on application-specific metrics, business metrics, or any other relevant metric that truly reflects your system’s performance and capacity needs.
The key components involved in custom metrics autoscaling are:
-
Metrics Server: A cluster-wide aggregator of resource usage data. It collects metrics from the Summary API, exposed by Kubelet on each node.
-
Custom Metrics API: An API that allows you to expose custom metrics to Kubernetes. This API is implemented by monitoring systems like Prometheus.
-
Prometheus Adapter: A popular tool that implements the custom metrics API and allows you to use Prometheus metrics for scaling decisions.
-
Horizontal Pod Autoscaler: Configured to use these custom metrics for scaling decisions.
The flow of information in a custom metrics autoscaling setup can be visualized as follows:
+----------------+ +-----------------+ +------------------+
| | | | | |
| Application |--->| Prometheus |--->| Prometheus |
| (Metrics | | (Scrapes & | | Adapter |
| Endpoint) | | Stores | | (Implements |
| | | Metrics) | | Custom Metrics |
| | | | | API) |
+----------------+ +-----------------+ +------------------+
|
|
v
+------------------+ +------------------+
| | | |
| Horizontal Pod |<---| Kubernetes API |
| Autoscaler | | Server |
| (Makes Scaling | | (Queries Custom |
| Decisions) | | Metrics) |
| | | |
+------------------+ +------------------+
This setup allows for highly flexible and application-specific scaling decisions, enabling you to scale based on metrics that truly reflect your application’s performance and capacity needs.
Implementing Custom Metrics Autoscaling in EKS
Now, let’s walk through the process of implementing custom metrics autoscaling in an EKS cluster. We’ll go through this step-by-step, explaining each component and its role in the autoscaling process.
Step 1: Set up the Metrics Server
The Metrics Server is a prerequisite for autoscaling. In EKS, you can deploy it using the following command:
kubectl apply -f https://github.com/kubernetes-sigs/metrics-server/releases/latest/download/components.yaml
This command deploys the Metrics Server in your cluster, which will start collecting CPU and memory usage metrics from all nodes and pods in the cluster.
Why it’s important: The Metrics Server provides the foundation for resource-based autoscaling. It aggregates resource usage data across your cluster, which is then used by the HPA for making scaling decisions.
Step 2: Deploy Prometheus
Prometheus is a popular open-source monitoring solution that integrates well with Kubernetes. You can deploy it using Helm:
helm repo add prometheus-community https://prometheus-community.github.io/helm-charts
helm repo update
helm install prometheus prometheus-community/prometheus
These commands add the Prometheus Helm repository, update the local repository cache, and install Prometheus in your cluster.
Why we need Prometheus: While the Metrics Server provides basic CPU and memory metrics, Prometheus allows us to collect and store custom application-specific metrics. This is crucial for implementing autoscaling based on business-relevant metrics.
Step 3: Deploy the Prometheus Adapter
The Prometheus Adapter allows Kubernetes to use Prometheus metrics for autoscaling decisions. Deploy it using Helm:
helm install prometheus-adapter prometheus-community/prometheus-adapter
This command installs the Prometheus Adapter, which will act as a bridge between Prometheus and the Kubernetes custom metrics API.
The role of Prometheus Adapter: It translates Prometheus metrics into a format that Kubernetes understands, making these metrics available for autoscaling decisions. This is what enables us to use application-specific metrics for scaling.
Certainly! I’ll continue from where we left off:
Step 4: Configure your application to expose custom metrics
Your application needs to expose metrics in a format that Prometheus can scrape. This typically involves adding a /metrics
endpoint to your application that exposes metrics in the Prometheus format. We’ll see a detailed example of this in our Go application demo later.
Why this step is crucial: By exposing custom metrics, you’re providing the raw data that will drive your autoscaling decisions. These metrics should be carefully chosen to reflect the actual load and performance of your application.
Step 5: Configure Prometheus to scrape your application
Add a scrape config to your Prometheus configuration:
scrape_configs:
- job_name: 'my-app'
kubernetes_sd_configs:
- role: pod
relabel_configs:
- source_labels: [__meta_kubernetes_pod_label_app]
regex: my-app
action: keep
This configuration tells Prometheus to scrape metrics from pods labeled with app: my-app
.
The importance of proper scraping: Ensuring that Prometheus is correctly scraping your application’s metrics is critical. Without this, your custom metrics won’t be available for autoscaling decisions.
Step 6: Create a HorizontalPodAutoscaler resource
Now you can create an HPA that uses your custom metric:
apiVersion: autoscaling/v2beta1
kind: HorizontalPodAutoscaler
metadata:
name: my-app-hpa
spec:
scaleTargetRef:
apiVersion: apps/v1
kind: Deployment
name: my-app
minReplicas: 1
maxReplicas: 10
metrics:
- type: Pods
pods:
metricName: http_requests_per_second
targetAverageValue: 1000m
This HPA will scale your application based on the average http_requests_per_second
across all pods, trying to maintain an average of 1000 milli-requests per second per pod.
Understanding the HPA configuration:
scaleTargetRef
: Specifies which deployment to scale.minReplicas
andmaxReplicas
: Set the scaling boundaries.metrics
: Defines which metric to use for scaling decisions.targetAverageValue
: The desired value of the metric. Here,1000m
means 1000 milli-requests, or 1 request per second.
Advanced Go Application Demo
Let’s create a more advanced Go application that not only exposes a custom metric but also simulates varying load conditions. This will help us better understand how our autoscaling setup responds to changing demand.
package main
import (
"fmt"
"math/rand"
"net/http"
"time"
"github.com/prometheus/client_golang/prometheus"
"github.com/prometheus/client_golang/prometheus/promhttp"
)
var (
httpRequestsTotal = prometheus.NewCounter(prometheus.CounterOpts{
Name: "http_requests_total",
Help: "Total number of HTTP requests",
})
httpRequestDuration = prometheus.NewHistogram(prometheus.HistogramOpts{
Name: "http_request_duration_seconds",
Help: "Duration of HTTP requests in seconds",
Buckets: prometheus.DefBuckets,
})
activeRequests = prometheus.NewGauge(prometheus.GaugeOpts{
Name: "http_requests_active",
Help: "Number of active HTTP requests",
})
)
func init() {
prometheus.MustRegister(httpRequestsTotal)
prometheus.MustRegister(httpRequestDuration)
prometheus.MustRegister(activeRequests)
}
func simulateWork() time.Duration {
// Simulate work that takes between 100ms and 500ms
workDuration := time.Duration(100+rand.Intn(400)) * time.Millisecond
time.Sleep(workDuration)
return workDuration
}
func handler(w http.ResponseWriter, r *http.Request) {
start := time.Now()
activeRequests.Inc()
defer activeRequests.Dec()
httpRequestsTotal.Inc()
duration := simulateWork()
httpRequestDuration.Observe(duration.Seconds())
fmt.Fprintf(w, "Hello, you're request number %d! It took %v\n", httpRequestsTotal, duration)
}
func main() {
http.HandleFunc("/", handler)
http.Handle("/metrics", promhttp.Handler())
fmt.Println("Server starting on :8080")
http.ListenAndServe(":8080", nil)
}
This application does the following:
- Imports necessary packages, including the Prometheus client library.
- Defines custom metrics:
http_requests_total
: A counter for the total number of requests.http_request_duration_seconds
: A histogram of request durations.http_requests_active
: A gauge of currently active requests.
- Registers these metrics with Prometheus.
- Implements a
simulateWork
function to mimic varying processing times. - Implements a handler function that:
- Increments the request counter
- Simulates work
- Records the request duration
- Updates the active requests gauge
- Sets up HTTP routes for the main handler and the
/metrics
endpoint.
To deploy this application in your EKS cluster, you’ll need to:
- Build the Docker image
- Push the image to a container registry (e.g., Amazon ECR)
- Create a Kubernetes Deployment for your application
Here’s an example Deployment manifest:
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-app
spec:
replicas: 1
selector:
matchLabels:
app: my-app
template:
metadata:
labels:
app: my-app
spec:
containers:
- name: my-app
image: your-repo/my-app:latest
ports:
- containerPort: 8080
resources:
requests:
cpu: 100m
memory: 128Mi
limits:
cpu: 500m
memory: 512Mi
This deployment starts with a single replica and sets resource requests and limits to ensure the pod has enough resources to run but doesn’t consume more than necessary.
Comprehensive Load Testing with k6
To truly understand how our autoscaling setup performs, we need to simulate real-world traffic patterns. For this, we’ll use k6, a modern load testing tool. Let’s create a more sophisticated k6 script that simulates varying levels of traffic:
import http from 'k6/http';
import { sleep } from 'k6';
export const options = {
scenarios: {
ramp_up_down: {
executor: 'ramping-vus',
startVUs: 0,
stages: [
{ duration: '5m', target: 100 }, // Ramp up to 100 users
{ duration: '10m', target: 100 }, // Stay at 100 for 10 minutes
{ duration: '5m', target: 200 }, // Ramp up to 200 users
{ duration: '10m', target: 200 }, // Stay at 200 for 10 minutes
{ duration: '5m', target: 0 }, // Ramp down to 0 users
],
gracefulRampDown: '2m',
},
},
};
export default function () {
const res = http.get('http://your-app-url/');
const requestDuration = res.timings.duration;
// Log the response time
console.log(`Response time: ${requestDuration}ms`);
// Simulate user think time
sleep(Math.random() * 3 + 1); // Random sleep between 1-4 seconds
}
This k6 script does the following:
- Defines a scenario that ramps up to 100 users, maintains that for 10 minutes, then ramps up to 200 users, maintains that for another 10 minutes, and finally ramps down to 0.
- For each virtual user, it makes a GET request to your application.
- It logs the response time for each request.
- It simulates user think time with a random sleep between requests.
To run this test:
- Save the script as
load_test.js
- Run the test with:
k6 run load_test.js
As the test runs, you should see your HPA in action, scaling your application up and down based on the defined metrics. You can monitor this using:
kubectl get hpa -w
This will show you real-time updates of your HPA’s status, including the current number of replicas and the values of the metrics it’s using for scaling decisions.
Best Practices and Architectural Considerations
When implementing custom metrics autoscaling, consider the following best practices:
-
Choose appropriate metrics: The metrics you choose should accurately reflect your application’s load and performance. CPU and memory usage are good starting points, but application-specific metrics like requests per second or queue length often provide better scaling signals.
-
Set realistic scaling thresholds: Start conservative and adjust based on observed behavior. Avoid setting thresholds too low, which can lead to unnecessary scaling events.
-
Implement proper readiness and liveness probes: These ensure that new pods are ready to receive traffic before old ones are scaled down, maintaining application availability during scaling events.
-
Use Pod Disruption Budgets: These help maintain application availability during voluntary disruptions, such as node drains during cluster upgrades.
-
Consider scaling cooldown periods: This prevents rapid fluctuations in the number of replicas, which can lead to instability.
-
Monitor and log extensively: Comprehensive monitoring and logging are crucial for understanding your application’s behavior and fine-tuning your autoscaling configuration.
-
Test thoroughly: Conduct load tests that simulate various traffic patterns to ensure your autoscaling setup responds appropriately under different conditions.
-
Plan for failure: Ensure your application can handle the sudden loss of instances, as this can happen during scale-down events.
-
Optimize your application for quick startup: The faster your application can start and become ready, the more responsive your autoscaling will be.
-
Use node affinity and pod anti-affinity: These can help distribute your application across different nodes or availability zones, improving resilience.
Troubleshooting and Performance Tuning
When working with custom metrics autoscaling, you may encounter various issues. Here are some common problems and how to address them:
-
Metrics not being collected:
- Check if Prometheus is correctly scraping your application.
- Verify that your application is exposing metrics correctly.
- Use
kubectl port-forward
to access Prometheus UI and check if your metrics are present.
-
HPA not scaling based on custom metrics:
- Ensure the Prometheus Adapter is correctly configured.
- Check if the custom metrics are available to Kubernetes:
kubectl get --raw "/apis/custom.metrics.k8s.io/v1beta1"
- Verify that the metric names in your HPA configuration match those exposed by your application.
-
Erratic scaling behavior:
- Review your HPA configuration, particularly the scaling thresholds.
- Consider implementing or adjusting cooldown periods to prevent rapid fluctuations.
- Analyze your application logs and metrics to understand what’s triggering the scaling events.
-
Performance degradation during scaling:
- Optimize your application’s startup time.
- Implement proper readiness probes to ensure traffic is only sent to ready pods.
- Consider using preemptive scaling for predictable traffic patterns.
-
Resource constraints preventing scaling:
- Check if your cluster has enough resources to accommodate new pods.
- Consider implementing the Cluster Autoscaler if you’re hitting node-level resource limits.
Remember, tuning your autoscaling configuration is an iterative process. Continuously monitor your application’s performance and scaling behavior, and be prepared to make adjustments as you learn more about your application’s behavior under different load conditions.
Future-proofing Your Autoscaling Strategy
As cloud-native technologies continue to evolve, it’s important to keep an eye on emerging trends and technologies that could impact your autoscaling strategy:
-
Machine Learning-based Autoscaling: Predictive autoscaling using machine learning models is an emerging trend. These models can learn from historical data to predict future resource needs and scale proactively.
-
Serverless and FaaS Integration: As serverless technologies mature, consider how they might complement your existing autoscaling strategy. Serverless functions can handle sudden spikes in traffic without the need for traditional autoscaling.
-
Service Mesh Integration: Service meshes like Istio provide advanced traffic management capabilities. Integrating your autoscaling with a service mesh can allow for more sophisticated scaling based on network-level metrics.
-
Multi-Cluster and Multi-Cloud Autoscaling: As organizations adopt multi-cloud strategies, autoscaling across multiple clusters or even multiple cloud providers is becoming more relevant.
-
Autoscaling for Stateful Applications: While most autoscaling focuses on stateless applications, advancements in this area are making it easier to autoscale stateful applications as well.
Stay informed about these trends and evaluate how they might benefit your specific use cases. Remember, the goal is always to create a system that’s resilient, efficient, and cost-effective.
Conclusion
Implementing custom metrics autoscaling in Amazon EKS is a powerful way to optimize your cluster’s performance and resource utilization. By following this guide, you’ve gained the knowledge to implement a sophisticated autoscaling strategy tailored to your application’s specific needs.
Remember that autoscaling is not a set-it-and-forget-it solution. It requires ongoing monitoring, tuning, and adjustment as your application evolves and your understanding of its behavior under different load conditions deepens.
As you continue to work with Kubernetes and EKS, keep exploring new tools and techniques. The cloud-native landscape is constantly evolving, and staying informed about new developments will help you build increasingly sophisticated and efficient systems.
Finally, always keep the end goal in mind: providing a seamless, responsive experience for your users while optimizing resource usage and costs. With the knowledge and tools you’ve gained from this guide, you’re well-equipped to tackle this challenge head-on. Happy scaling!