The Top 5 Caching Patterns Every Engineer Should Master
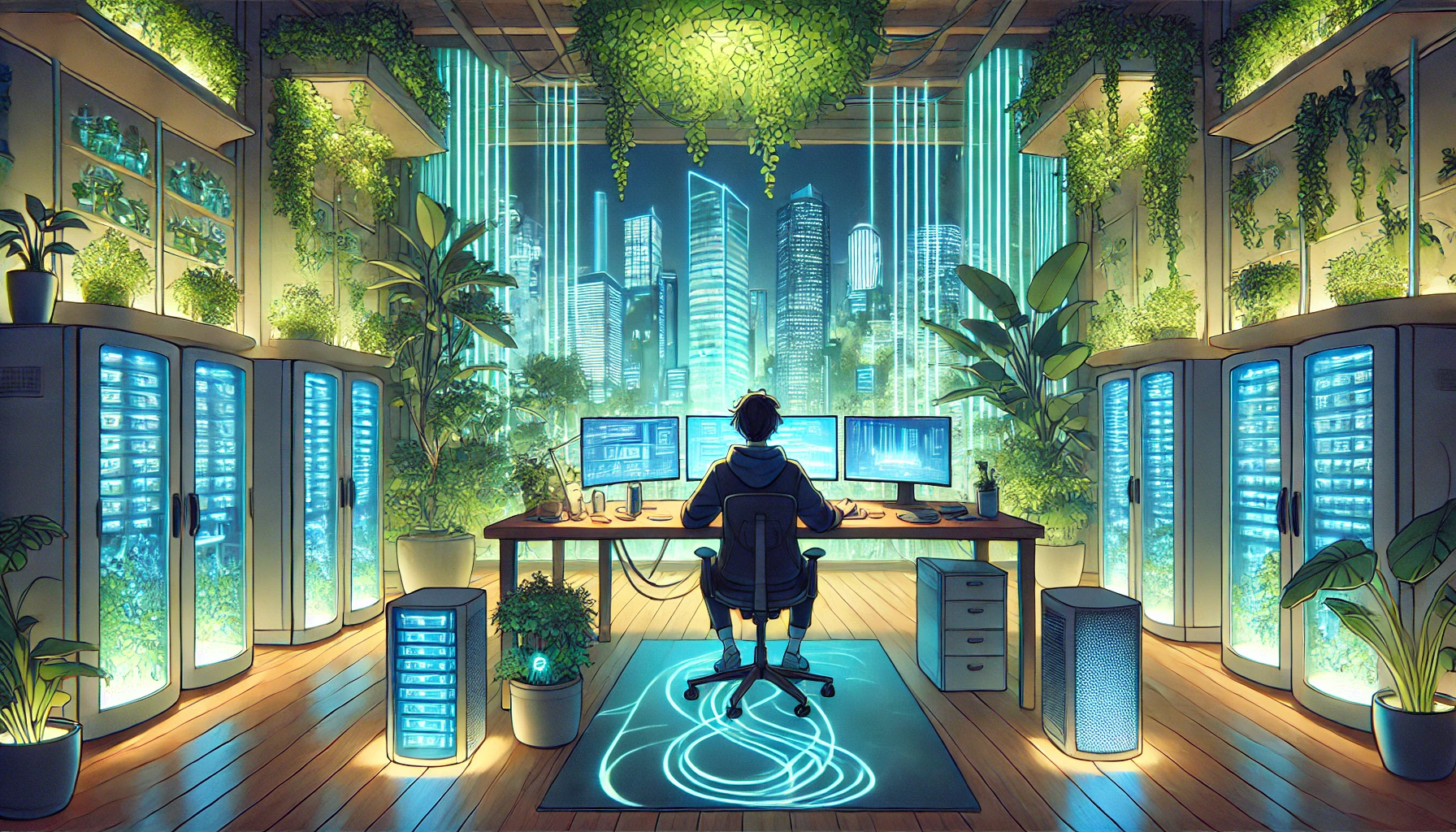
Caching is a cornerstone of efficient system design, enabling applications to deliver near-instant responses by temporarily storing frequently accessed data. However, “just store data in Redis” is rarely enough—effective caching requires nuanced approaches to consistency, latency, cache invalidation, and operational concerns like concurrency and scaling.
In this guide, we’ll explore the top five caching patterns, supplemented with extended Go examples using Redis. Whether you’re optimizing a distributed microservices architecture or fine-tuning a single monolithic application, these patterns will give you the knowledge to build robust, high-performance, and scalable systems.
Table of Contents
- Introduction to Caching
- Understanding Cache Hits and Misses
- Top 5 Caching Patterns
- Choosing the Right Caching Strategy
- Go Implementation Examples
- Best Practices and Considerations
- Conclusion
- References
Introduction to Caching
At its core, caching involves storing copies of data in a fast-access storage layer (the cache) so that subsequent requests can be served more quickly than if they were retrieved from a slower datastore like a disk-based database or an external API. The most common caching layers include:
- In-memory data stores: e.g., Redis, Memcached
- In-process cache: e.g., local memory or language-specific cache libraries
- CDNs (Content Delivery Networks): for static assets or partially dynamic content
Why Caching Matters
- Performance and Latency: In-memory lookups are orders of magnitude faster than disk or network I/O, drastically reducing response times.
- Scalability: By offloading read traffic from your primary datastore or external services, you can handle higher loads with fewer resources.
- Reduced Costs: Serving data from cache can reduce the number of expensive database queries or downstream API calls.
Key Challenges
- Data Freshness: Ensuring the cached data is up to date, especially in write-intensive or real-time systems.
- Invalidation Complexity: Determining when and how to invalidate stale data in the cache.
- Concurrency and Race Conditions: Handling multiple writes or reads to the same data safely.
These challenges are not insurmountable but require well-structured caching patterns to solve elegantly.
Understanding Cache Hits and Misses
Two fundamental metrics guide caching effectiveness:
- Cache Hit: Data is found in the cache. This is the best-case scenario: low latency, minimal load on the downstream datastore.
- Cache Miss: Data is absent from the cache, forcing a fetch from the primary datastore (DB or external API). This incurs higher latency.
Your cache hit ratio—the percentage of requests that result in hits—directly impacts your system’s performance. For read-heavy applications, a higher hit ratio can substantially cut response times. For systems with more balanced reads and writes, consistency and write performance might trump raw hit ratio.
Top 5 Caching Patterns
Below are five commonly used caching patterns. Each has different trade-offs regarding latency, data consistency, operational complexity, and memory usage. Selecting the correct pattern for your scenario is crucial.
1. Cache Aside
Also known as Lazy Loading, Cache Aside is among the simplest and most widely used approaches.
Workflow
-
Read Request
- Application → Cache: Attempt to read from cache.
- If cache hit, return the data.
- If cache miss, fetch data from the database (or external store), store it in the cache, then return the data.
-
Write Request
- Write data directly to the DB.
- Optionally invalidate or update the cache so subsequent reads reflect the new data.
[Client] --> [Application] --> (Check) --> [Cache]
| Miss
V
[Database]
Advantages
- Simplicity: Minimal code changes and conceptual overhead.
- On-Demand Caching: Only the data that’s accessed gets cached, reducing memory footprint.
Disadvantages
- Stale Data Risk: If you don’t proactively invalidate the cache, stale data can linger until it expires.
- Miss Penalty: For a popular key, sudden spikes in concurrent requests can lead to repeated cache misses (the “thundering herd” problem).
Use Cases
- General-Purpose Read-Heavy: Product catalogs, user profiles, microservices retrieving reference data.
- Infrequent Writes: Systems where updates are relatively rare but reads are frequent.
2. Write Through
In Write Through, the cache is the first point of write and is always kept in sync with the database.
Workflow
-
Write Request
- The application writes data to the cache.
- The cache immediately writes the data to the underlying datastore synchronously.
-
Read Request
- Reads are served directly from the cache, which is guaranteed to have the latest data.
[Client] --> [Application] --> [Cache] --> [Database]
Advantages
- Strong Consistency: The cache is never out of date relative to the database.
- Fast Reads: Because all data is always up to date in the cache, reads have low latency.
Disadvantages
- Slower Writes: Writes involve both the cache and the database in one operation, doubling the overhead.
- Cache Pollution: Data that’s written but rarely read will still live in the cache, using up memory.
Use Cases
- Critical Consistency: Financial transactions, inventory management for e-commerce, or any domain where stale reads are unacceptable.
- Moderate to Low Write Frequency: The overhead of synchronous database writes can be acceptable if writes are less frequent than reads.
3. Read Through
Read Through functions similarly to Cache Aside, but the caching layer (or a dedicated library) takes responsibility for fetching data on a miss, rather than the application code.
Workflow
-
Read Request
- Application → Cache: If data is present, return it immediately.
- If cache miss, the cache mechanism itself fetches data from the DB, stores it, and returns it to the application.
-
Write Request
- Typically written directly to the DB (or a combined write-through approach in some libraries).
- The cache can then invalidate or update itself automatically.
[Read Flow]
[Client] --> [Application] --> [Cache "layer"]
| (If miss)
V
[Database]
Advantages
- Encapsulation: Caching details are abstracted away behind a library or proxy, reducing duplication of cache-hit/miss logic in your code.
- Transparent to Application: Developers can “treat” the cache like the main datastore for reads.
Disadvantages
- Implementation Complexity: Out-of-the-box solutions might be easy if your framework supports them, but rolling your own can be tricky.
- Possible Data Staleness: Careful invalidation or TTLs are still required to avoid returning stale data.
Use Cases
- High-Read Environments: E-commerce product pages, subscription content, streaming metadata.
- Where Developers Want Minimal Cache Logic: The library or proxy “just handles” caching for you.
4. Write Back
Also called Write-Behind, Write Back optimizes for write performance by deferring the actual database update.
Workflow
-
Write Request
- Application → Cache: Immediately write data to the cache and return success to the client.
- The cache then asynchronously flushes (or batches) changes to the database after a short delay or based on some policy.
-
Read Request
- Reads come from the cache, which holds the newest data.
[Client] --> [Application] --> [Cache]
(Asynchronous flush)
|
V
[Database]
Advantages
- Extremely Fast Writes: The system returns faster responses to write operations since the database write is deferred.
- Batching & Reduced IOPS: You can batch multiple updates and perform them in a single operation, potentially lowering I/O overhead.
Disadvantages
- Risk of Data Loss: If the cache node fails before the data is flushed, changes are lost.
- Conflict Complexity: Handling conflicts or partial failures in the background can be complex, especially in distributed systems.
Use Cases
- Write-Heavy Workloads: Real-time analytics, logging, telemetry, IoT data ingestion.
- Eventual Consistency Acceptance: Systems where losing a small amount of data is tolerable or data is quickly re-generated.
5. Write Around
Write Around eschews updating the cache on writes. Instead, the application writes directly to the database, and the cache is only updated on subsequent reads.
Workflow
-
Write Request
- Application → Database: Data is stored in the DB.
- Cache is not updated at this time (or optionally invalidated).
-
Read Request
- Application → Cache: If data is not present, fetch it from the DB and populate the cache.
[Client] --> [Application] --> [Database]
|
| (Cache filled on read)
V
[Cache]
Advantages
- Less Cache Pollution: You don’t store data in the cache if it might never be read.
- Direct DB Writes: Provides immediate durability for new or updated data.
Disadvantages
- More Frequent Misses: If the data is read frequently soon after being written, the cache will miss until the read populates it.
- Inconsistent Performance: The first read after a write is slower, especially if your DB is under high load.
Use Cases
- Sporadically Accessed Data: Configuration, metadata, or other items read infrequently.
- Large Datasets: When caching everything is prohibitively expensive, and you only want hot data in cache.
Choosing the Right Caching Strategy
Selecting a caching pattern requires balancing performance, consistency, operational complexity, and hardware constraints:
- Read vs. Write Mix:
- Write-Heavy: Consider Write Back for speed or Write Through for consistent data.
- Read-Heavy: Cache Aside, Read Through, or Write Around can reduce read latency.
- Consistency Requirements:
- Strict Consistency: Write Through ensures data in cache and DB is always in sync.
- Eventual Consistency: Write Back might suffice if some delay is acceptable.
- Cache Pollution vs. Hit Ratio:
- If memory is expensive or limited, patterns like Write Around help avoid caching rarely read data.
- If memory is abundant, Write Through ensures everything is in cache for quick reads.
- Operational Complexity & Failures:
- Write Back requires a robust mechanism for async flushing; losing cache data might mean losing writes.
- Cache Aside is conceptually simpler but can suffer from stale data if you forget to invalidate.
Go Implementation Examples
Below are practical Go/Redis examples demonstrating each caching pattern. These examples assume:
- You’ve installed and are running Redis locally (or via Docker).
- You have the Go Redis client installed (
github.com/go-redis/redis/v9
). - You understand the basics of Go concurrency and error handling.
Setting Up Redis with Go
# Run Redis via Docker
docker run --name redis -p 6379:6379 -d redis
# Install the Go Redis client
go get github.com/go-redis/redis/v9
Initialize your Redis client:
package main
import (
"context"
"fmt"
"time"
"strings"
"strconv"
"github.com/go-redis/redis/v9"
)
var ctx = context.Background()
func initializeRedis() *redis.Client {
rdb := redis.NewClient(&redis.Options{
Addr: "localhost:6379",
Password: "",
DB: 0,
})
if _, err := rdb.Ping(ctx).Result(); err != nil {
panic(err)
}
return rdb
}
func main() {
rdb := initializeRedis()
// Use rdb in subsequent functions
// ...
}
This sets up a Redis client ready for use in your application.
Implementing Cache Aside in Go
Scenario: We have user profiles that are read frequently but updated occasionally.
type User struct {
ID string
Name string
Email string
}
// Cache Aside: getUserProfile
func getUserProfile(rdb *redis.Client, userID string) (*User, error) {
// Attempt to read from Redis cache
val, err := rdb.Get(ctx, userID).Result()
if err == redis.Nil {
// Cache miss, fetch from DB
user, err := fetchUserFromDB(userID)
if err != nil {
return nil, err
}
// Store the fetched data in Redis with a TTL
userData := fmt.Sprintf("%s:%s:%s", user.ID, user.Name, user.Email)
if err := rdb.Set(ctx, userID, userData, 10*time.Minute).Err(); err != nil {
return nil, err
}
return &user, nil
} else if err != nil {
// Some other Redis error
return nil, err
}
// Cache hit: parse the data
parts := strings.Split(val, ":")
return &User{
ID: parts[0],
Name: parts[1],
Email: parts[2],
}, nil
}
// Simulated DB fetch
func fetchUserFromDB(userID string) (User, error) {
// In a real scenario, query your database here
// We'll just pretend we did
return User{
ID: userID,
Name: "John Doe",
Email: "[email protected]",
}, nil
}
// Cache Aside: updateUserProfile
func updateUserProfile(rdb *redis.Client, user User) error {
// Write to DB first
if err := updateUserInDB(user); err != nil {
return err
}
// Invalidate or update the cache
// Option 1: Just delete the key
if err := rdb.Del(ctx, user.ID).Err(); err != nil {
return err
}
// Option 2: Or set the new data immediately
// userData := fmt.Sprintf("%s:%s:%s", user.ID, user.Name, user.Email)
// if err := rdb.Set(ctx, user.ID, userData, 10*time.Minute).Err(); err != nil {
// return err
// }
return nil
}
// Simulated DB update
func updateUserInDB(user User) error {
fmt.Printf("DB update: user %s changed to Name=%s, Email=%s\n", user.ID, user.Name, user.Email)
return nil
}
Tip: To avoid a “thundering herd” on a popular key, consider using a distributed lock (like Redlock) to ensure that only one process fetches and updates the cache at a time.
Implementing Write Through in Go
Scenario: Manage inventory levels in an e-commerce system where strong consistency is important.
// Write Through: updateInventory
func updateInventory(rdb *redis.Client, itemID string, quantity int) error {
// Start a Redis pipeline or transaction
pipe := rdb.TxPipeline()
// Write to cache first
pipe.Set(ctx, itemID, quantity, 0)
// Synchronously write to DB in the same operation
_, err := pipe.Exec(ctx, func() error {
// Attempt DB update
if dbErr := updateInventoryInDB(itemID, quantity); dbErr != nil {
return dbErr
}
return nil
})
return err
}
// Simulated DB write
func updateInventoryInDB(itemID string, quantity int) error {
fmt.Printf("DB updated: item %s has quantity %d\n", itemID, quantity)
return nil
}
// Write Through: getInventory
func getInventory(rdb *redis.Client, itemID string) (int, error) {
val, err := rdb.Get(ctx, itemID).Result()
if err == redis.Nil {
// If data somehow isn't in cache (it should be), revert to DB
quantity, err := fetchInventoryFromDB(itemID)
if err != nil {
return 0, err
}
// Restore to cache so subsequent reads are fast
if err := rdb.Set(ctx, itemID, quantity, 0).Err(); err != nil {
return 0, err
}
return quantity, nil
} else if err != nil {
return 0, err
}
// Parse quantity
qty, _ := strconv.Atoi(val)
return qty, nil
}
func fetchInventoryFromDB(itemID string) (int, error) {
// Simulated DB lookup
return 100, nil // default to some quantity
}
Note: In real-world usage, you might structure the transaction logic differently (e.g., manually checking for errors before committing the pipeline), but the principle remains the same.
Implementing Read Through in Go
Scenario: A high-traffic product catalog where reads are extremely frequent, and you want minimal boilerplate code in your application logic.
type Product struct {
ID string
Name string
Price float64
}
// Read Through: getProductDetails
func getProductDetails(rdb *redis.Client, productID string) (*Product, error) {
val, err := rdb.Get(ctx, productID).Result()
if err == redis.Nil {
// Cache miss
product, err := fetchProductFromDB(productID)
if err != nil {
return nil, err
}
// Store in Redis
productData := fmt.Sprintf("%s:%s:%f", product.ID, product.Name, product.Price)
if err := rdb.Set(ctx, productID, productData, 5*time.Minute).Err(); err != nil {
return nil, err
}
return &product, nil
} else if err != nil {
return nil, err
}
// Cache hit
parts := strings.Split(val, ":")
price, _ := strconv.ParseFloat(parts[2], 64)
return &Product{
ID: parts[0],
Name: parts[1],
Price: price,
}, nil
}
func fetchProductFromDB(productID string) (Product, error) {
// Simulated DB fetch
return Product{
ID: productID,
Name: "Gadget Pro",
Price: 299.99,
}, nil
}
In some frameworks, a true “read-through” might mean the caching system automatically fetches from the DB on a miss without explicit code. The above snippet demonstrates the concept in a more manual form.
Implementing Write Back in Go
Scenario: High-speed analytics or real-time data ingestion where write performance is paramount, and eventual consistency (plus minor data loss risk) is acceptable.
// Write Back: logUserActivity
func logUserActivity(rdb *redis.Client, userID, activity string) error {
activityData := fmt.Sprintf("%s:%s", userID, activity)
// Immediately write to cache (e.g., a list or stream)
// LPush will store new entries at the head of the list
if err := rdb.LPush(ctx, "user_activity_log", activityData).Err(); err != nil {
return err
}
// Asynchronously flush to DB
go func() {
// This is a simple single-event flush, but you could batch multiple writes
if err := writeActivityToDB(userID, activity); err != nil {
// Optionally add retry logic, dead-letter queues, or logs
fmt.Printf("DB write failed: %v\n", err)
}
}()
return nil
}
func writeActivityToDB(userID, activity string) error {
// Simulated DB write
fmt.Printf("Persisted user %s activity: %s\n", userID, activity)
return nil
}
In practice, you might batch writes periodically (e.g., every few seconds) to reduce DB overhead. You also need a robust strategy to handle node failures—persisting data frequently or replicating your cache layer.
Implementing Write Around in Go
Scenario: Rarely accessed configuration settings. When a new setting is saved, it goes straight to DB and the cache is only updated if someone reads it later.
// Write Around: updateConfigSetting
func updateConfigSetting(rdb *redis.Client, configKey, configValue string) error {
// Write to DB
if err := updateConfigInDB(configKey, configValue); err != nil {
return err
}
// Optionally invalidate the cache if it might have old data
if err := rdb.Del(ctx, configKey).Err(); err != nil {
return err
}
return nil
}
func getConfigSetting(rdb *redis.Client, configKey string) (string, error) {
val, err := rdb.Get(ctx, configKey).Result()
if err == redis.Nil {
// Cache miss, fetch from DB
configValue, err := fetchConfigFromDB(configKey)
if err != nil {
return "", err
}
// Populate cache for subsequent reads
if err := rdb.Set(ctx, configKey, configValue, 30*time.Minute).Err(); err != nil {
return "", err
}
return configValue, nil
} else if err != nil {
return "", err
}
// Cache hit
return val, nil
}
// Simulated DB operations
func updateConfigInDB(configKey, configValue string) error {
fmt.Printf("DB updated config: %s = %s\n", configKey, configValue)
return nil
}
func fetchConfigFromDB(configKey string) (string, error) {
// Return a placeholder value
return "Enabled", nil
}
This approach keeps your cache lean but at the cost of potential cache misses soon after a write.
Best Practices and Considerations
-
Cache Invalidation
- Decide whether to evict keys manually (on writes) or rely on time-based TTLs. Incorrect or missing invalidation can lead to serving stale data.
-
Monitoring & Metrics
- Track cache hit ratio, latency (both cache latency and DB fallback), and key eviction rates. Low hit ratios may indicate suboptimal TTLs or that your workload is ill-suited to caching.
-
Serialization & Compression
- Data stored in Redis is typically serialized (JSON, Gob, Protobuf). Large objects may benefit from compression to reduce memory usage at the cost of CPU overhead.
-
Eviction Policies
- Redis supports LRU, LFU, volatile-ttl, and more. Choose based on how you want to remove old or least-used data when memory is constrained.
-
High Availability
- Use Redis Sentinel or Redis Cluster for automatic failover, or replicate data if downtime is unacceptable. For extremely high durability, consider combining Redis with persistent stores.
-
Security
- Secure Redis with strong passwords, TLS, or network isolation. Sensitive data in cache might need encryption at rest or in transit.
-
Concurrency Controls
- Race conditions on read-write or write-write can cause inconsistencies. Tools like Redlock help orchestrate distributed locks.
- For patterns like Write Back, robust queueing or transaction logs reduce data loss from crashes.
-
Thundering Herd Mitigation
- For very popular keys, implement strategies like request coalescing, exponential backoff, or lock-based fetch to prevent dozens of concurrent cache misses from hammering your database simultaneously.
Conclusion
Caching can be a game-changer in system design, drastically improving performance, cost efficiency, and scalability. However, the choice of which caching pattern you employ is just as critical as the decision to cache at all:
- Cache Aside (Lazy Loading): Great for read-heavy workloads with occasional writes.
- Write Through: Ensures data in cache is always consistent, at the cost of write performance.
- Read Through: Abstracts read logic into a library or proxy, reducing code complexity.
- Write Back (Write-Behind): Maximizes write speed but requires careful handling of data durability.
- Write Around: Avoids cache pollution by only caching data on demand after a read.
Each pattern carries unique trade-offs around concurrency, data freshness, memory usage, and operational complexity. As you design or refine your system’s caching layer, keep in mind the importance of monitoring, invalidation strategies, concurrency controls, and reliability measures (like replication or persistent logs). By aligning your caching strategy with your application’s workload and consistency requirements, you’ll unlock the full power of caching to build resilient, scalable, and high-performance systems.
References
- Prisma - Introduction to Database Caching
- From Cache to In-Memory Data Grid: Introduction to Hazelcast
- Alachisoft - ReadThrough, WriteThrough, WriteBehind Caching Patterns
- Go-Redis Documentation
- Redis Official Documentation
Follow me on LinkedIn and Twitter for more system design insights, caching best practices, and Go programming tips.
Want deeper dives? Subscribe to my newsletter for hands-on design case studies, caching tutorials, and more Go-based architectures.